Introduction
ASCII art is a fascinating way to represent visual images using the characters from the ASCII (American Standard Code for Information Interchange) character set. From creating stunning visuals with simple text to adding retro flair to your designs ASCII art has both creative and practical applications.
In this blog post, we’ll explore what ASCII art is, why it’s useful, and how you can transform images into ASCII art using Python.
What Is ASCII Art?
At its core, ASCII art is a technique that recreates images using text characters such as letters, numbers, and symbols. Each character corresponds to a certain level of brightness or density, which allows text to mimic the appearance of an image.
For example, characters like @
, #
, and %
are used for darker areas, while lighter spaces are represented by characters like ., -, or a blank space. When arranged correctly, these characters create an illusion of depth and texture that represents the original image in a text-based format.
1ლ(╹◡╹ლ) ASCII ART2
3 @4 @@@5 @@@@@6 @@@@@@@@@@@7 @@@@@8 |||9 |||10 ___|||___11 // \\12 || ||13 ||_________||14 \\_______//
ASCII art has been popular in tech culture for decades, from early computer systems that lacked graphical interfaces to modern tools and creative projects.
Transform Images to ASCII Art with Python
ASCII art is a fascinating way to represent visual images using the characters from the ASCII (American Standard Code for Information Interchange) character set. From creating stunning visuals with simple text to adding retro flair to your designs, ASCII art has both creative and practical applications.
In this blog post, we’ll explore what ASCII art is, why it’s useful, and how you can transform images into ASCII art using Python.
What Is ASCII Art? At its core, ASCII art is a technique that recreates images using text characters such as letters, numbers, and symbols. Each character corresponds to a certain level of brightness or density, which allows text to mimic the appearance of an image.
For example, characters like @, #, and % are used for darker areas, while lighter spaces are represented by characters like ., -, or a blank space. When arranged correctly, these characters create an illusion of depth and texture that represents the original image in a text-based format.
ASCII art has been popular in tech culture for decades, from early computer systems that lacked graphical interfaces to modern tools and creative projects.
Why Is ASCII Art Useful?
While ASCII art may seem like just a fun artistic experiment, it has a variety of real-world uses:
-
Retro Aesthetic: ASCII art evokes a nostalgic charm reminiscent of early computing and terminal-based systems. It's often used in retro-style designs, games, and art.
-
File Size Optimization: Unlike images, ASCII art is made up entirely of plain text, making it lightweight and easy to share across platforms with limited resources or bandwidth.
-
Compatibility: Since ASCII art is just text, it can be displayed on any device or platform that supports text, even in environments where images aren't supported.
-
Creative Experimentation: ASCII art opens up unique possibilities for creativity, allowing developers, artists, and hobbyists to explore new ways to represent visual content.
-
Terminal Graphics: For developers working in terminal environments, ASCII art can be a playful and functional way to display information or enhance user interfaces.
How to Transform Images into ASCII Art with Python?
Python, with its simplicity and rich ecosystem of libraries, makes it easy to transform images into ASCII art. Here's a brief outline of the process:
-
Convert Image to Grayscale: Images in grayscale represent brightness values more clearly, which are essential for mapping characters to image pixels.
-
Map Brightness to Characters: Define a character set where darker characters (e.g., @, #, %) represent higher intensity and lighter characters (e.g., ., -, ) represent lower intensity.
-
Resize the Image: To maintain proportions and avoid distortion, resize the image while keeping the width-to-height ratio in mind.
-
Generate the ASCII Art: Replace each pixel in the image with a corresponding character based on its brightness value.
-
Display or Save the Art: The final ASCII art can be printed in the console, saved as a text file, or even displayed as HTML.
A Simple Python Implementation
Here’s a quick Python script to get you started with ASCII art:
A safer and more isolated approach is to create a virtual environment. This allows you to install packages like pillow without affecting the system Python installation.
a) Create a virtual environment
1python3 -m venv myenv
b) Activate the virtual environment
1source myenv/bin/activate
c) Install dependencies inside the virtual environment
1pip install pillow
d) Run your script within the virtual environment
1python your_script.py
You need to install those dependencies:
1pip install pillow2pip install image
And here is the complete script:
1from PIL import Image2
3# Define the ASCII characters in descending order of brightness4ASCII_CHARS = "@%#*+=-:. " # Length of this string is 105
6def image_to_ascii(image_path, new_width=50): # Default width reduced for smaller images7 # Open the image and convert it to grayscale8 image = Image.open(image_path).convert("L")9
10 # Resize the image while maintaining the aspect ratio11 aspect_ratio = image.height / image.width12 new_height = int(new_width * aspect_ratio * 0.55) # Adjust height scaling (smaller factor)13 image = image.resize((new_width, new_height))14
15 # Map pixels to ASCII characters16 pixels = image.getdata()17 ascii_str = ''.join([ASCII_CHARS[int(pixel / 255 * (len(ASCII_CHARS) - 1))] for pixel in pixels])18
19 # Split the ASCII string into rows20 ascii_art = [ascii_str[i:i+new_width] for i in range(0, len(ascii_str), new_width)]21 return "\n".join(ascii_art)22
23# Test the function24image_path = "/Users/your_user/Downloads/maxim-berg-QNVh6QXPXLk-unsplash.jpg" # Replace with your image path25ascii_art = image_to_ascii(image_path, new_width=50) # Adjust the width for smaller output26print(ascii_art)27
28# Optional: Save ASCII art to a file29with open("ascii_art.txt", "w") as file:30 file.write(ascii_art)
Example output
Explore some tests of the script and sample images from unsplash.
Note that this is some low quality scanning, and adjusting the size and the display font size prameters allow You to make more detailed scans of the image.
@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@@@@%##****#%@@@@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@#=-:::::::::-+%@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@#+:..::::::::::::-*%@@@@@@@@@@@@@@ @@@@@@@@@@@@@@#=:.:-:::-=++++++=---+#%@@@@@@@@@@@@ @@@@@@@@@@@@*=:.:=*-:=#%%%%%##*++-==+##@@@@@@@@@@@ @@@@@@@@@@%-.:=+**--=*#%#+=+##*-+-=*+=+*%@@@@@@@@@ @@@@@@@@@#-.+%%%*--++##*=+*++***#*+=+=+++@@@@@@@@@ @@@@@@@@%=:+%%%*-:*#*+=-==++==*+=-+==+*++#@@@@@@@@ @@@@@@@@#+*#%%#=:*#*:....:-----::-+++**++#@@@@@@@@ @@@@@@@%####%%+:*%%%#****+==-----++**#*+##@@@@@@@@ @@@@@@@#**-+%#=*%%###*+#%%#*+=---:--+*+**%#@@@@@@@ @@@@@@*++++*#%%%%%###*==*%%%#*=--=+*##+++##%@@@@@@ @@@@@%*****-=%%%%%%%#*+==*#%%%####*###*+=+*#@@@@@@ @@@@@%*+##**##%%%%###*+=++*#*#%#***********#@@@@@@ @@@@@%+**+++*=**%%###*+++++**#####*++*+++#*#@@@@@@ @@@@@@%*+*+**+++*%#***+++++++#%%%*--=++*###%@@@@@@ @@@@@@@@%#*##++*+**========--==++=+*#**++*#%@@@@@@ @@@@@@@@@#**#***+++++++++++==-==++**##*++**%@@@@@@ @@@@@@@@@@#*#####*+*+***+************##***#@@@@@@@ @@@@@@@@@@%*#%##%#*+**#****+*************%@@@@@@@@ @@@@@@@@@@@%*##*###*++##***+++*******#%%@@@@@@@@@@ @@@@@@@@@@@@%*#**##*+++++++++*******#@@@@@@@@@@@@@ @@@@@@@@@@@@@##+*#****++=---=*##+-*+*@@@@@@@@@@@@@ @@@@@@@@@@@@@%#+*#*+*#**=---=#%#=-+**@@@@@@@@@@@@@ @@@@@@@@@@@@@##++*++###*=-=-=#%#==+**@@@@@@@@@@@@@ @@@@@@@@@@@@%*#=+*+=*###+==-+##*-+***@@@@@@@@@@@@@ @@@@@@@@@@#=+*#==++=*%%%*===*###+*+**@@@@@@@@@@@@@ @@@@@@@@%-::-*#===+=*%%%#*=+#%###*+**@@@@@@@@@@@@@ @@@@@@@#==**+**=====+#%%%*+*%%#%*+++*@@@@@@@@@@@@@ @@@@@@@*++**#**=====+##%##**#%%#++++*@@@@@@@@@@@@@ @@@@@@@@%##****++===+####****##*++=+#@@@@@@@@@@@@@ @@@@@@@@@@@@@%##*++++*##%%##***++++#@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@%##%%@@@@@@@%**+*#%@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@ @@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@
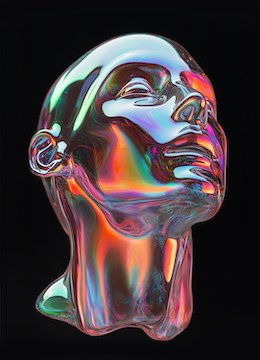
Styling of pre for ASCII art
Below You will find the css styles to display the <pre></pre>
element properly for Your generated ASCII Art.
1pre.ascii {2 font-family: "Courier New", Courier, monospace; /* Use a monospaced font */3 white-space: pre-line; /* Preserve whitespace and line breaks */4 overflow: auto; /* Allow scrolling if content overflows */5 background-color: #111111; /* Dark background for contrast */6 color: #00ff00; /* Green text for a classic terminal look */7 padding: 1rem; /* Add padding for better readability */8 border-radius: 8px; /* Slightly rounded corners */9 font-size: 12px;10 letter-spacing: 0;11 line-height: 1;12 max-width: 393px; /* Prevent overflow beyond container */13 box-shadow: 0 4px 6px rgba(0, 0, 0, 0.2); /* Add a subtle shadow */14 text-wrap: wrap;15}
Conclusion
ASCII art transforms ordinary images into creative, text-based visuals that are not only nostalgic but also practical in many scenarios. Whether you're creating something fun for your terminal, adding a retro vibe to your projects, or exploring the intersection of art and code, ASCII art is an excellent way to experiment.
With Python, the process of generating ASCII art is accessible and highly customizable. So grab your favorite image, run the script, and start creating your own ASCII masterpieces!
Have you tried making ASCII art before? Share your results or ideas in the comments below!
Comments
You must be logged in to comment.
Loading comments...