Introduction
In today's world, securing your property is more important than ever. Building a DIY laser security system using an Arduino UNO offers an affordable and customizable solution to protect your home or office. This project leverages automation and hardware components to create an effective monitoring system that alerts you to any unauthorized entry.
Requirements
To build your DIY laser security system, you will need the following components and tools:
- Arduino UNO
- Laser Module
- Photoresistor (LDR)
- Resistors x 6 (e.g., 10kΩ)
- Breadboard
- Jumper Wires
- LED Indicators x 4
- Buzzer x 1
- Button x 1
- USB Cable for Arduino
- Computer with Arduino IDE installed
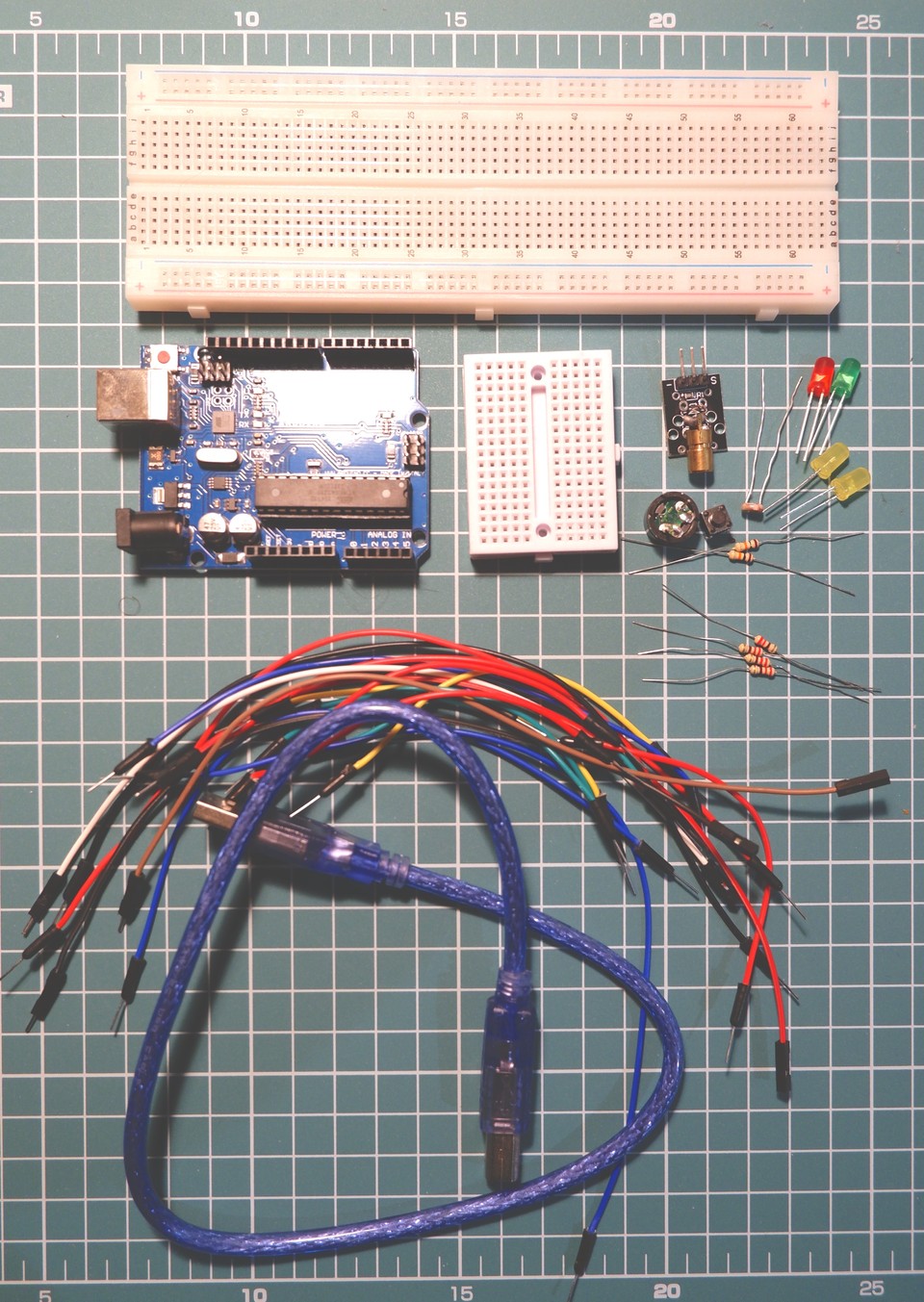
Setup of Pins and Components
Wiring Setup
-
Laser Module Connection:
- Connect the positive lead of the laser module to pin 5V on the Arduino UNO.
- Connect the negative lead to the GND (ground) pin.
-
Photoresistor (LDR) Connection:
- Connect one end of the photoresistor to A0 on the Arduino.
- Connect the other end to A0 (Analog Pin 0) through a 10kΩ resistor.
- Connect the junction between the photoresistor and resistor to A0.
-
LED Indicator and Buzzer:
- Connect the LEDs positive leads to pins 4, 5, 6, 8 through a resistor.
- Connect the Buzzer positive lead to pin 12.
- Both components' negative leads should be connected to GND.
Pin Configuration Table
1| Component | Arduino Pin |2| ------------- | ----------- |3| Laser Module | 5V GND |4| Photoresistor | A0 |5| Red LED | 4 |6| Green LED | 5 |7| Arming Button | 6 |8| Yellow LED | 7 |9| Yellow LED2 | 8 |10| Buzzer | 12 |
Diagram and Components Wiring
Technical drawing explanation of the setup.
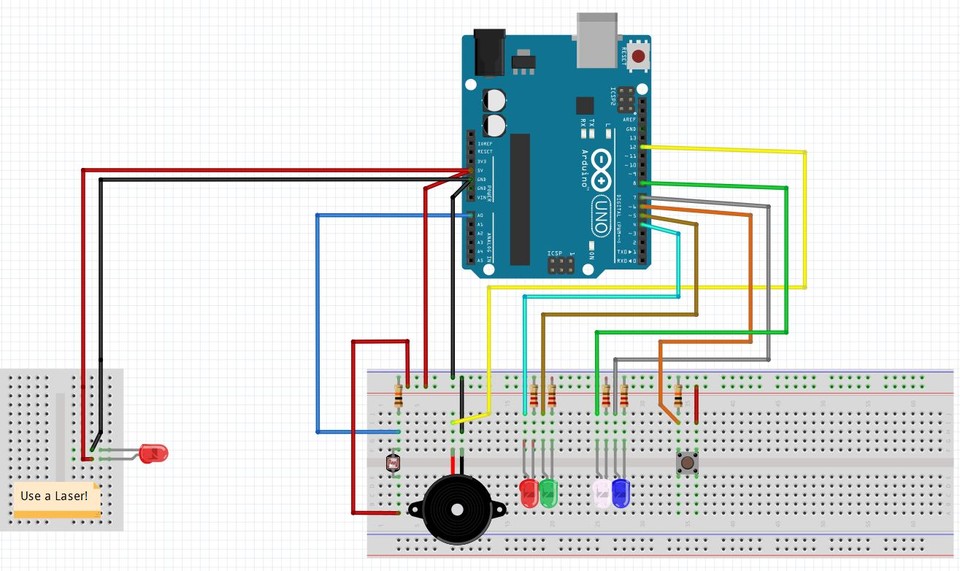
Below is the full components wiring setup photo.
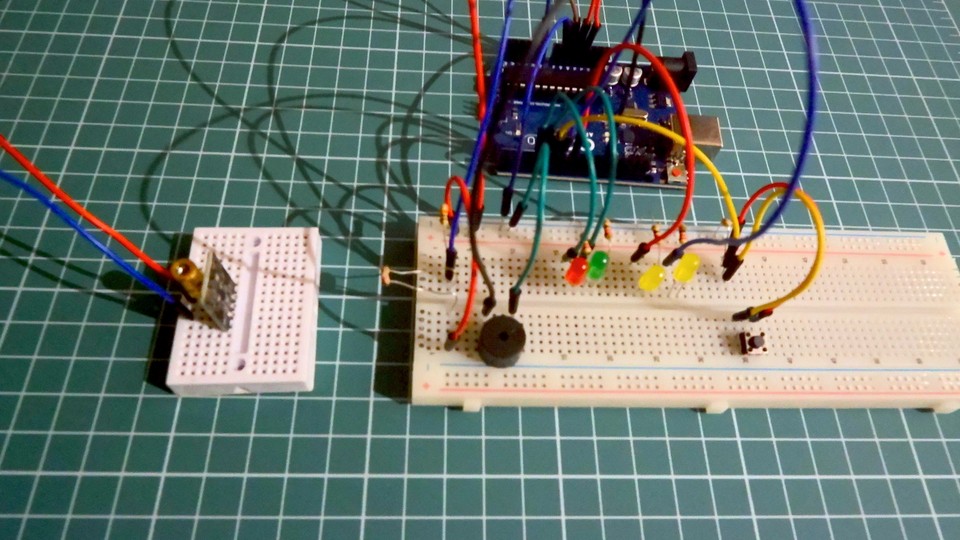
Coding the Arduino Board
Below is the Arduino code for the laser security system. This code reads input from the photoresistor and controls the laser accordingly.
1// Arduino code for laser security system2const int triggeredLED = 7;3const int triggeredLED2 = 8;4const int RedLED = 4;5const int GreenLED = 5;6const int inputPin = A0;7const int speakerPin = 12;8const int armButton = 6;9
10boolean isArmed = true;11boolean isTriggered = false;12int buttonVal = 0;13int prev_buttonVal = 0;14int reading = 0;15int threshold = 0;16
17
18const int lowrange = 2000;19const int highrange = 4000;20
21void setup(){22
23 Serial.begin(9600);24 pinMode(triggeredLED, OUTPUT);25 pinMode(triggeredLED2, OUTPUT);26 pinMode(RedLED, OUTPUT);27 pinMode(GreenLED, OUTPUT);28 pinMode(armButton, INPUT);29 digitalWrite(triggeredLED, HIGH);30 delay(500);31 digitalWrite(triggeredLED, LOW);32
33 calibrate();34 setArmedState();35}36
37void loop(){38
39
40 reading = analogRead(inputPin);41 //Send data to the Serial Port42 Serial.println(reading);43 int buttonVal = digitalRead(armButton);44 if ((buttonVal == HIGH) && (prev_buttonVal == LOW)){45 setArmedState();46 delay(500);47 }48
49 if ((isArmed) && (reading < threshold)){50 isTriggered = true;}51
52 if (isTriggered){53
54 for (int i = lowrange; i <= highrange; i++)55 {56 tone (speakerPin, i, 150);57 }58
59 for (int i = highrange; i >= lowrange; i--)60 {61 tone (speakerPin, i, 150);62 }63
64
65 digitalWrite(triggeredLED, HIGH);66 delay(50);67 digitalWrite(triggeredLED, LOW);68 delay (50);69 digitalWrite(triggeredLED2, HIGH);70 delay (50);71 digitalWrite(triggeredLED2, LOW);72 delay (50);73 }74
75 delay(20);76}77
78void setArmedState(){79
80 if (isArmed){81 digitalWrite(GreenLED, HIGH);82 digitalWrite(RedLED, LOW);83 isTriggered = false;84 isArmed = false;85 } else {86 digitalWrite(GreenLED, LOW);87 digitalWrite(RedLED, HIGH);88 tone(speakerPin, 220, 125);89 delay(200);90 tone(speakerPin, 196, 250);91 isArmed = true;92 }93}94
95void calibrate(){96
97 int sample = 0;98 int baseline = 0;99 const int min_diff = 200;100 const int sensitivity = 50;101 int success_count = 0;102
103 digitalWrite(RedLED, LOW);104 digitalWrite(GreenLED, LOW);105
106 for (int i=0; i<10; i++){107 sample += analogRead(inputPin);108 digitalWrite(GreenLED, HIGH);109 delay (50);110 digitalWrite(GreenLED, LOW);111 delay (50);112 }113
114 do115 {116 sample = analogRead(inputPin);117
118 if (sample > baseline + min_diff){119 success_count++;120 threshold += sample;121
122 digitalWrite(GreenLED, HIGH);123 delay (100);124 digitalWrite(GreenLED, LOW);125 delay (100);126 } else {127 success_count = 0;128 threshold = 0;129 }130
131 } while (success_count < 3);132
133 threshold = (threshold/3) - sensitivity;134
135 tone(speakerPin, 196, 250);136 delay(200);137 tone(speakerPin, 220, 125);138}
Code Explanation
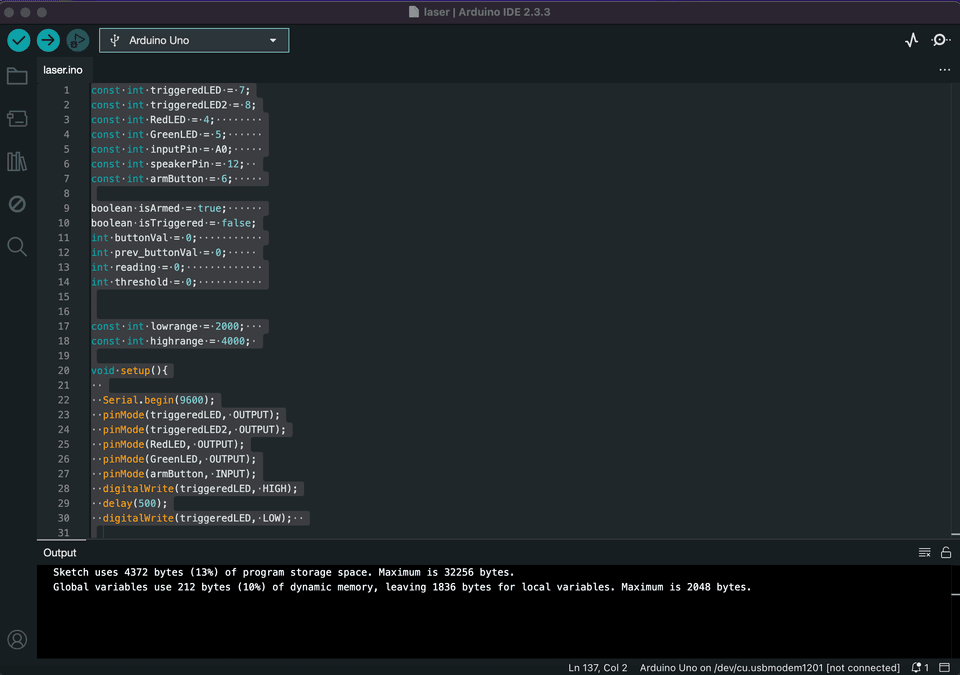
Variables Declaration
Pin Assignments
triggeredLED
&triggeredLED2
: LEDs that flash when the system is triggered.RedLED
: Indicates the system is armed.GreenLED
: Indicates the system is disarmed.inputPin
: Reads analog data from the photoresistor.speakerPin
: Emits audible alarms via a piezo speaker.armButton
: Button used to toggle between armed and disarmed states.
State Variables
isArmed
: Boolean to track if the system is armed.isTriggered
: Boolean to track if the system has been triggered.buttonVal
&prev_buttonVal
: Track the arm button state.reading
: Current sensor reading.threshold
: Sensitivity level for triggering the alarm.
Constants for Tone Ranges
lowrange
&highrange
: Frequency range for the alarm sound.
Functions Overview
Setup Function
- Serial Communication: Initializes serial communication at 9600 baud for debugging.
- Pin Modes: Configures LEDs, the button, and speaker pins as
OUTPUT
orINPUT
appropriately. - Calibration: Calls the
calibrate
function to set the initial threshold based on the environment. - Armed State Initialization: Calls
setArmedState
to initialize the system to an armed or disarmed state.
Loop Function
-
Sensor Reading:
- Continuously reads the sensor value (
reading
) from the photoresistor and logs it to the serial monitor.
- Continuously reads the sensor value (
-
Button Press Handling:
- Toggles the armed/disarmed state when the arm button is pressed.
-
Trigger Check:
- If the system is armed (
isArmed
) and the sensor value drops below the threshold, the alarm is triggered (isTriggered
).
- If the system is armed (
-
Alarm Activation:
- When triggered, the following actions are performed:
- The speaker emits a sweeping tone (frequency oscillation between
lowrange
andhighrange
). - The LEDs (
triggeredLED
andtriggeredLED2
) blink alternately to indicate the breach.
- The speaker emits a sweeping tone (frequency oscillation between
- When triggered, the following actions are performed:
setArmedState Function
- Toggles the armed state of the system:
- If Armed:
- Lights the green LED.
- Turns off the red LED.
- Resets the triggered state.
- If Disarmed:
- Lights the red LED.
- Turns off the green LED.
- Plays a disarm tone sequence on the speaker.
- If Armed:
calibrate Function
- Calibration Setup:
- Collects a baseline reading over 10 samples while blinking the green LED.
- Threshold Adjustment:
- Monitors the sensor and adjusts the threshold when a significant change (greater than
min_diff
) is detected for 3 consecutive readings.
- Monitors the sensor and adjusts the threshold when a significant change (greater than
- Final Threshold Calculation:
- Averages the significant readings and subtracts the
sensitivity
constant to set the final threshold value.
- Averages the significant readings and subtracts the
- Calibration Completion:
- Plays a tone sequence to indicate successful calibration.
Workflow Summary
- System Initialization:
- The system calibrates itself and initializes the armed state.
- Arming/Disarming:
- The user can toggle the system's armed state using the button.
- Monitoring:
- Continuously reads sensor values and checks against the threshold.
- Alarm Triggering:
- Activates visual and audible alarms when the laser beam is blocked (sensor reading drops below the threshold).
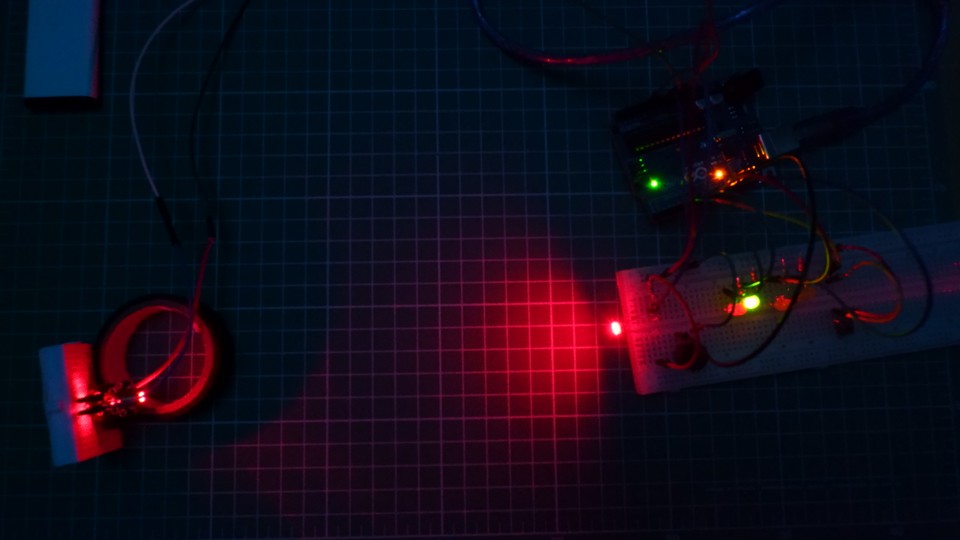
Testing
To ensure your updated laser security system operates correctly, follow these testing steps:
-
Component Verification:
- Check all connections against the wiring diagram.
- Use a multimeter to verify voltage levels where applicable.
-
System Activation:
- Upload the updated Arduino code to the UNO board.
- Power the system and verify the calibration process:
- The green LED should blink during calibration.
- A tone sequence should play on the speaker upon successful calibration.
- Ensure the red LED is lit to indicate the system is armed.
-
Simulating Intrusion:
- Block the laser beam by placing an object in its path.
- If the system is armed:
- Observe the
triggeredLED
andtriggeredLED2
alternately flashing. - Listen for the sweeping tone from the speaker.
- Observe the
- Check the serial monitor for sensor value changes and ensure they fall below the threshold when the laser beam is interrupted.
-
Arming/Disarming:
- Press the arm button to toggle the system state:
- In the armed state, the red LED should light up, and the system should monitor for intrusions.
- In the disarmed state, the green LED should light up, and the system should ignore intrusions.
- Press the arm button to toggle the system state:
-
Threshold Adjustment:
- If the system is too sensitive or not sensitive enough, adjust the
sensitivity
ormin_diff
values in the calibration section of the code. - Re-upload the code and retest until optimal performance is achieved.
- If the system is too sensitive or not sensitive enough, adjust the
-
Troubleshooting:
- System Not Arming/Disarming: Verify connections to the
armButton
pin and ensure the button is functional. - No Sensor Reading: Check the photoresistor wiring and resistor values.
- Speaker Not Sounding: Ensure the speaker is connected to the correct pin (
speakerPin
) and functioning. - LEDs Not Responding: Verify the connections to
triggeredLED
,triggeredLED2
,RedLED
, andGreenLED
pins.
- System Not Arming/Disarming: Verify connections to the
By following these steps, you can ensure the system operates as intended and performs reliably under various conditions.
Conclusion
Building a DIY laser security system with an Arduino UNO is an excellent project for enthusiasts interested in automation and hardware programming. This project not only enhances your security setup but also deepens your understanding of sensor integration and Arduino coding. Future enhancements could include adding wireless notifications, integrating with smartphones, or expanding the system to monitor multiple entry points. Experiment with different configurations and components to tailor the system to your specific security needs.
Comments
You must be logged in to comment.
Loading comments...